ECMAScript 6的支持还在开发中,已经支持了es6的大部分特性。
性能好:它将JavaScript编译为.NET字节码(CIL),而非解释执行。这样使得它的运行性能更好;
纯.Net开发:纯.Net开发,不依赖任何第三方库,只有单个dll文件,可以完全集成到.Net框架中,可以在任何支持.Net平台执行;
使用:
1,可以直接用 NuGet 获取 Jurassic。
using Jurassic;
简单的执行js字符串
var engine = new Jurassic.ScriptEngine();
engine.Evaluate("function main(a,b){return a+b;}");
var addResult= engine.CallGlobalFunction("main", 5, 6);//结果11
engine.Evaluate("function main(a,b){return a+b;}");
var addResult= engine.CallGlobalFunction("main", 5, 6);//结果11
2,.Net 和 javascpript 之间交互。
暴露net的class给js
暴露net的class的静态方法
暴露net的类实例
[复制到剪贴板] |
using Jurassic;
using Jurassic.Library;
public class AppInfo : ObjectInstance
{
public AppInfo(ScriptEngine engine)
: base(engine)
{
// 重写name属性
this["name"] = "Test Application";
// 只读属性
this.DefineProperty("version", new PropertyDescriptor(5, PropertyAttributes.Sealed), true);
}
}
engine.SetGlobalValue("appInfo", new AppInfo(engine));
Console.WriteLine(engine.Evaluate<string>("appInfo.name + ' ' + appInfo.version"));
暴露net的class的静态方法
[复制到剪贴板] |
using Jurassic;
using Jurassic.Library;
public class Math2 : ObjectInstance
{
public Math2(ScriptEngine engine)
: base(engine)
{
this.PopulateFunctions();
}
[JSFunction(Name = "log10")]
public static double Log10(double num)
{
return Math.Log10(num);
}
}
engine.SetGlobalValue("math2", new Math2(engine));
engine.Evaluate<double>("math2.log10(1000)");
暴露net的类实例
[复制到剪贴板] |
using Jurassic;
using Jurassic.Library;
public class RandomConstructor : ClrFunction
{
public RandomConstructor(ScriptEngine engine)
: base(engine.Function.InstancePrototype, "Random", new RandomInstance(engine.Object.InstancePrototype))
{
}
[JSConstructorFunction]
public RandomInstance Construct(int seed)
{
return new RandomInstance(this.InstancePrototype, seed);
}
}
public class RandomInstance : ObjectInstance
{
private Random random;
public RandomInstance(ObjectInstance prototype)
: base(prototype)
{
this.PopulateFunctions();
this.random = new Random(0);
}
public RandomInstance(ObjectInstance prototype, int seed)
: base(prototype)
{
this.random = new Random(seed);
}
[JSFunction(Name = "nextDouble")]
public double NextDouble()
{
return this.random.NextDouble();
}
}
engine.SetGlobalValue("Random", new RandomConstructor(engine));
engine.Evaluate<double>("var rand = new Random(1000); rand.nextDouble()");
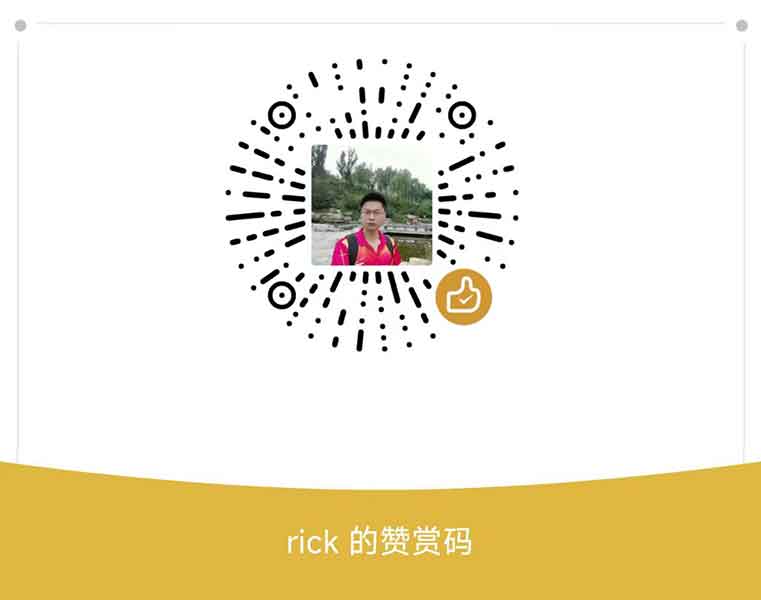